动手学深度学习-39
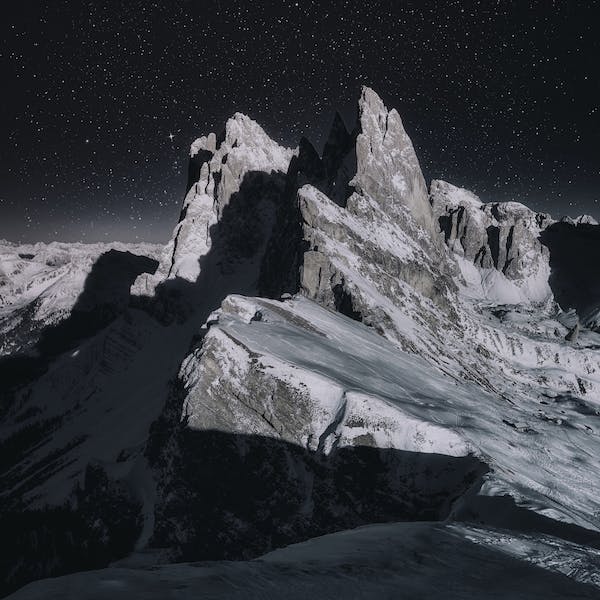
实战Kaggle比赛,图像分类(CIFAR-10)
这里将从原始图像文件开始,然后逐步组织、读取并将它们转换为张量格式
获取并组织数据集
比赛数据集分为训练集和测试集,其中训练集包含50000张、测试集包含300000张图像。在测试集中,10000张图像将被用于评估,而剩下的290000张图像将不会被进行评估,包含它们只是为了防止手动标记测试集并提交标记结果(防作弊)。两个数据集中的图像都是png格式,高度和宽度均为32像素并有三个颜色通道(RGB)。这些图片共涵盖10个类别:飞机、汽车、鸟类、猫、鹿、狗、青蛙、马、船和卡车。
下载数据集
为了便于入门,使用包含前1000个训练图像和5个随机测试图像的数据集的小规模样本。要使用Kaggle竞赛的完整数据集,需要将以下demo
变量设置为False
。
1 | import collections |
整理数据集
需要整理数据集来训练和测试模型。首先,我们用以下函数读取CSV文件中的标签,它返回一个字典,该字典将文件名中不带扩展名的部分映射到其标签。
1 | #@save |
定义reorg_train_valid
函数来将验证集从原始的训练集中拆分出来。此函数中的参数valid_ratio
是验证集中的样本数与原始训练集中的样本数之比。更具体地说,令valid_ratio=0.1
为例,由于原始的训练集有50000张图像,因此train_valid_test/train
路径中将有45000张图像用于训练,而剩下5000张图像将作为路径train_valid_test/valid
中的验证集。组织数据集后,同类别的图像将被放置在同一文件夹下。
1 | #@save |
下面的reorg_test
函数用来在预测期间整理测试集,以方便读取
1 | #@save |
最后,我们使用一个函数来调用前面定义的函数read_csv_labels
、reorg_train_valid
和reorg_test
1 | def reorg_cifar10_data(data_dir, valid_ratio): |
在这里,我们只将样本数据集的批量大小设置为32。在实际训练和测试中,应该使用Kaggle竞赛的完整数据集,并将batch_size
设置为更大的整数,例如128。我们将10%的训练样本作为调整超参数的验证集。
1 | batch_size = 32 if demo else 128 |
图像增广
我们使用图像增广来解决过拟合的问题。例如在训练中,我们可以随机水平翻转图像。我们还可以对彩色图像的三个RGB通道执行标准化。下面,我们列出了其中一些可以调整的操作。
1 | transform_train = torchvision.transforms.Compose([ |
读取数据集
接下来,读取由原始图像组成的数据集,每个样本都包括一张图片和一个标签
1 | train_ds, train_valid_ds = [torchvision.datasets.ImageFolder(os.path.join(data_dir, "train_valid_test", folder), transform=transform_train) for folder in ["train", "train_valid"]] |
在训练期间,我们需要指定上面定义的所有图像增广操作。当验证集在超参数调整过程中用于模型评估时,不应引入图像增广的随机性。
在最终预测之前,我们根据训练集和验证集组合而成的训练模型进行训练,以充分利用所有标记的数据。
1 | train_iter, train_valid_iter = [torch.utils.data.DataLoader(dataset, batch_size, shuffle=True, drop_last=True) for dataset in (train_ds, train_valid_ds)] |
定义模型
1 | def get_net(): |
定义训练函数
1 | # lr_period表示每隔多少时间(epoch)将lr减小, lr_decay表示lr减小多少(decay * lr) |
训练和验证模型
1 | devices, num_epochs, lr, wd = d2l.try_all_gpus(), 20, 2e-4, 5e-4 |
在 Kaggle 上对测试集进行分类并提交结果
在通过验证集调得具有超参数的满意的模型后,我们使用所有标记的数据(包括验证集)来重新训练模型并对测试集进行分类。
1 | net, preds = get_net(), [] |
小结
- 将包含原始图像文件的数据集组织为所需格式后,我们可以读取它们。
- 我们可以在图像分类竞赛中使用卷积神经网络和图像增广。
- 标题: 动手学深度学习-39
- 作者: 敖炜
- 创建于 : 2023-09-03 17:08:15
- 更新于 : 2024-04-19 09:29:16
- 链接: https://ao-wei.github.io/2023/09/03/动手学深度学习-39/
- 版权声明: 本文章采用 CC BY-NC-SA 4.0 进行许可。